01->New line
To insert a new line in the program , you need to end the current line using \n manipulator.
e.g
#include <iostream>
using namespace std;
int main()
{
cout << "hello world" \n;
cout << "C++ is very easy";
return 0;
}
02->Blank line
To insert a new line in the program , you need to end the current line using \n\n manipulator.
#include <iostream>
using namespace std;
int main()
{
cout << "hello world" \n\n;
cout << "C++ is very easy";
return 0;
}
Output:-
03->Multiple blank lines
#include <iostream>
using namespace std;
int main()
{
cout << "hello \n world \n ";
cout << "C++ \n programming \n is very\n easy";
return 0;
}
Output:-
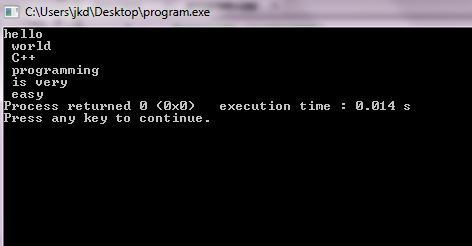
04-Variables
Variable are used in C++, where we need storage for any value, which will change in program. Variable can be declared in multiple ways....
EXAMPLE 1-printing a variable
#include <iostream>
using namespace std;
int main ()
{
int x = 23;
cout << "hello world \n";
cout << "my name is jatinder\n";
cout << "i am \n";
cout<< x;
cout<< "year old";
return 0;
}
Output:-
Note:-The integer can be declared in either way and you will get the same result..
int x = 23;
or
int x;
x=23;
also in the code, The varialbes shall never be written in quotation marks,, becase if you will type
cout << "x" ;
then it will type the letter x, and not the variable x
EXAMPLE 2- Adding two variables
#include <iostream>
using namespace std;
int main ()
{
int a=50;
int b=25;
int result = a+b;
cout << result;
return 0;
}
in above example we predefined the value of the variable..but if we want user to select a value for that variable, we use cin with an extraction operator( >>)
note that cout is written with insersion operator (<<)
Example 3- Doubling a number given by user
#include <iostream>
using namespace std;
int main ()
{
int a;
cout<< "please enter a numbers to be doubled \n ";
cin>> a;
cout<< a*2;
return 0;
}
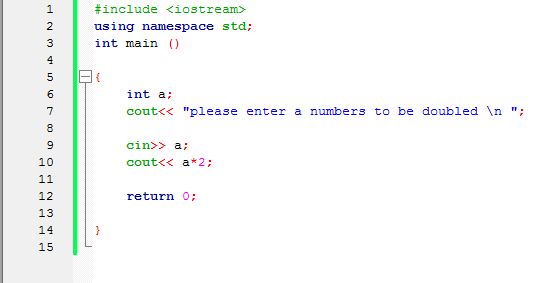
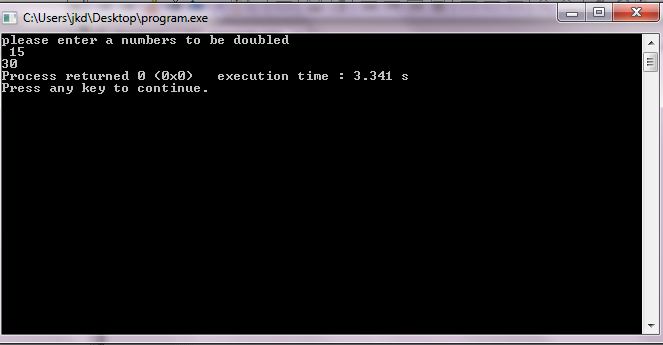
Example 4- accepting multiple inputs from user
In the next post, you will learn about conditional statements..
Conditional statements
To insert a new line in the program , you need to end the current line using \n manipulator.
e.g
#include <iostream>
using namespace std;
int main()
{
cout << "hello world" \n;
cout << "C++ is very easy";
return 0;
}
02->Blank line
To insert a new line in the program , you need to end the current line using \n\n manipulator.
#include <iostream>
using namespace std;
int main()
{
cout << "hello world" \n\n;
cout << "C++ is very easy";
return 0;
}
Output:-
03->Multiple blank lines
#include <iostream>
using namespace std;
int main()
{
cout << "hello \n world \n ";
cout << "C++ \n programming \n is very\n easy";
return 0;
}
Output:-
04-Variables
Variable are used in C++, where we need storage for any value, which will change in program. Variable can be declared in multiple ways....
EXAMPLE 1-printing a variable
#include <iostream>
using namespace std;
int main ()
{
int x = 23;
cout << "hello world \n";
cout << "my name is jatinder\n";
cout << "i am \n";
cout<< x;
cout<< "year old";
return 0;
}
Output:-
Note:-The integer can be declared in either way and you will get the same result..
int x = 23;
or
int x;
x=23;
also in the code, The varialbes shall never be written in quotation marks,, becase if you will type
cout << "x" ;
then it will type the letter x, and not the variable x
EXAMPLE 2- Adding two variables
#include <iostream>
using namespace std;
int main ()
{
int a=50;
int b=25;
int result = a+b;
cout << result;
return 0;
}
in above example we predefined the value of the variable..but if we want user to select a value for that variable, we use cin with an extraction operator( >>)
note that cout is written with insersion operator (<<)
Example 3- Doubling a number given by user
#include <iostream>
using namespace std;
int main ()
{
int a;
cout<< "please enter a numbers to be doubled \n ";
cin>> a;
cout<< a*2;
return 0;
}
Example 4- accepting multiple inputs from user
#include
using namespace std;
int main ()
{
int a,b,c;
cout<<"please enter a number"<
cin>>a;
cout<<"please enter another number"<
cin>>b;
c=a+b;
cout<<"the sum of two numbers is"<
return 0;
}
using namespace std;
int main ()
{
int a,b,c;
cout<<"please enter a number"<
cin>>a;
cout<<"please enter another number"<
cin>>b;
c=a+b;
cout<<"the sum of two numbers is"<
return 0;
}
In the next post, you will learn about conditional statements..
Conditional statements
No comments:
Post a Comment